Tutorial of PageTransition¶
PageTransition class has the aggregated log as the member variable and has the method functions to get the information.
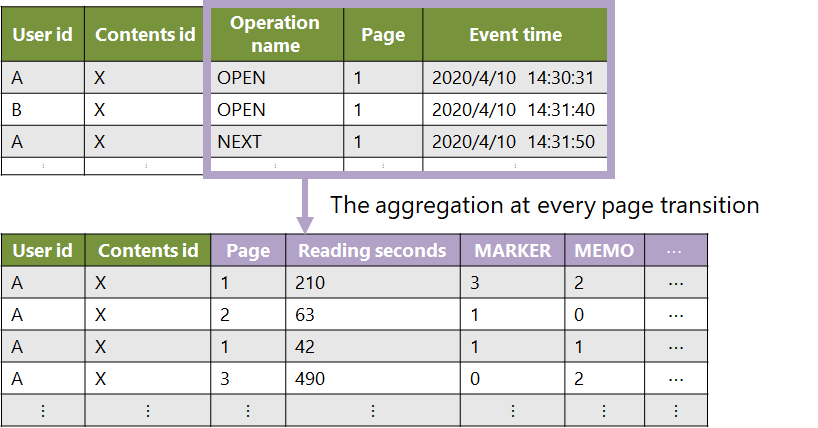
import openLA as la
operations = ["NEXT", "PREV", "ADD MARKER"]
course_info, event_stream = la.start_analysis(files_dir="dataset_sample", course_id="A")
operation_count = la.convert_into_page_transition(event_stream,
invalid_seconds=10,
timeout_seconds=30*60,
user_id=event_stream.user_id()[:10],
contents_id=event_stream.contents_id()[0],
operation_name=operations,
count_operation=True
)
The method functions in below table are available now.
You can get the information of the log like
page_transition.num_users()
.The documentation is in Page-wise Aggregation Document
function |
description |
---|---|
num_users |
Get the number of users in the log |
user_id |
Get the unique user ids in the log |
contents_id |
Get the unique contents ids in the log |
operation_name |
Get the unique operation name in the log |
operation_count |
Get the count of each (or specified) operation in the log |
reading_seconds |
Get the reading seconds in the log |
reading_time |
Get the reading time (seconds, minutes, or hours) in the log |
num_unique_pages |
Get the number of unique pages in the log |
unique_pages |
Get the unique page numbers in the log |
num_transition |
Get the number of transition in the log |
If you want to process other than the above functions, you can get DataFrame type event stream by page_transition.df
and process with Pandas library.
import OpenLA as la
import pandas as pd
operations = ["NEXT", "PREV", "ADD MARKER"]
course_info, event_stream = la.start_analysis(files_dir="dataset_sample", course_id="A")
page_transition = la.convert_into_page_transition(event_stream,
invalid_seconds=10,
timeout_seconds=30*60,
user_id=event_stream.user_id()[:10],
contents_id=event_stream.contents_id()[0],
operation_name=operations,
count_operation=True
)
page_transition_df = page_transition.df
print(page_transition_df)
"""
userid contentsid pageno reading_seconds time_of_entry time_of_exit NEXT PREV ADD MARKER
0 U1 C1 1 14 2018-04-08 17:53:47 2018-04-08 17:54:01 1 0 0
1 U1 C1 2 20 2018-04-08 17:54:01 2018-04-08 17:54:21 1 0 0
2 U1 C1 1 22 2018-04-10 15:00:06 2018-04-10 15:00:28 1 0 0
3 U1 C1 2 11 2018-04-10 15:00:28 2018-04-10 15:00:39 1 0 0
... ... ... ... ... ...
"""