Tutorial of TimeRangeAggregation¶
TimeRangeAggregation class has the aggregated log as the member variable and has the method functions to get the information.
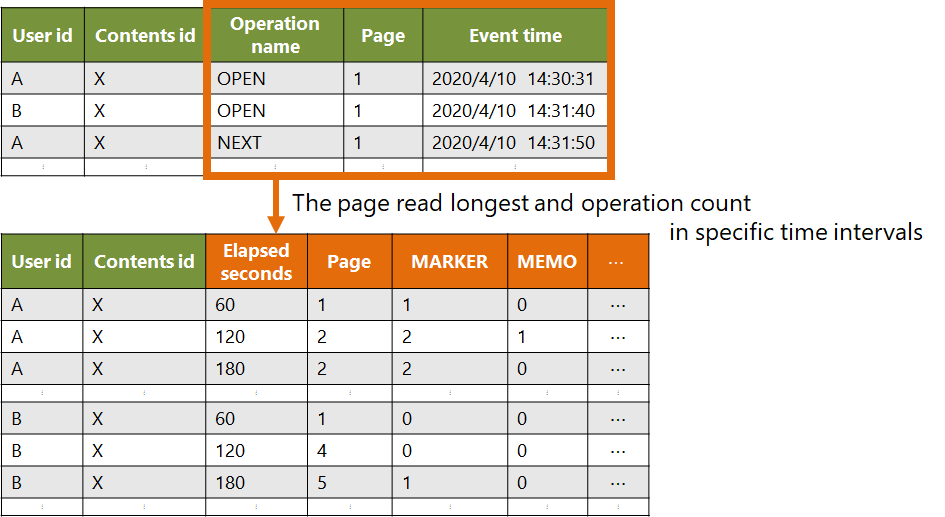
import OpenLA as la
import pandas as pd
operations = ["NEXT", "PREV", "ADD MARKER"]
course_info, event_stream = la.start_analysis(files_dir="dataset_sample", course_id="A")
user = event_stream.user_id()[5]
lecture = 1
content = "C1"
timerange_aggregation = la.convert_into_time_range(course_info, event_stream,
user_id=user,
contents_id=content,
lecture_week=lecture,
interval_seconds=60,
start_time='start_of_lecture',
end_time='end_of_lecture',
time_range_basis='minutes',
count_operation=True,
operation_name=operations,
)
timerange_df = timerange_aggregation.df
print(timerange_df)
"""
elapsed_minutes start_of_range end_of_range userid contentsid pageno NEXT PREV
0 1.0 2018-04-10 14:50:00 2018-04-10 14:51:00 U1 C1 1 0.0 0.0
1 2.0 2018-04-10 14:51:00 2018-04-10 14:52:00 U1 C1 1 0.0 0.0
2 3.0 2018-04-10 14:52:00 2018-04-10 14:53:00 U1 C1 1 0.0 0.0
3 4.0 2018-04-10 14:53:00 2018-04-10 14:54:00 U1 C1 1 0.0 0.0
4 5.0 2018-04-10 14:54:00 2018-04-10 14:55:00 U1 C1 1 0.0 0.0
.. ... ... ... ... ... ... ... ...
85 86.0 2018-04-10 16:15:00 2018-04-10 16:16:00 U1 C1 27 18.0 2.0
86 87.0 2018-04-10 16:16:00 2018-04-10 16:17:00 U1 C1 40 0.0 0.0
87 88.0 2018-04-10 16:17:00 2018-04-10 16:18:00 U1 C1 38 0.0 22.0
88 89.0 2018-04-10 16:18:00 2018-04-10 16:19:00 U1 C1 9 5.0 13.0
89 90.0 2018-04-10 16:19:00 2018-04-10 16:20:00 U1 C1 14 19.0 0.0
"""
The method functions in below table are available now.
You can get the information of the log like
timerange_aggregation.num_users()
.The documentation is in Time-range Aggregation Document
function |
description |
---|---|
num_users |
Get the number of users in the log |
user_id |
Get the unique user ids in the log |
contents_id |
Get the unique contents ids in the log |
operation_name |
Get the unique operation name in the log |
operation_count |
Get the count of each (or specified) operation in the log |
reading_seconds |
Get the reading seconds in the log |
num_unique_pages |
Get the number of unique pages in the log |
unique_pages |
Get the unique page numbers in the log |
If you want to process other than the above functions, you can get DataFrame type event stream by timerange_aggregation.df
and process with Pandas library.